CS 161 Lab B - Turtle Graphics
Due Fri Jan 30 at the start of class
Overview
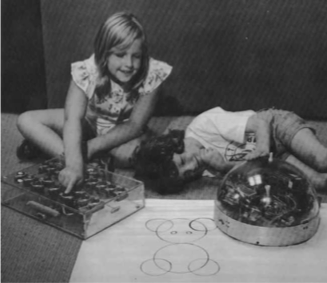
In the late 60s, Seymour Papert at MIT developed a robot "turtle" to help teach young children to program. This robot has a small pen attached to it, which would draw a trail (a line) on a piece of paper as the robot moved and turned. Children could then program the robot's movement in order to have it draw a particular picture. This method of producing drawings is known as turtle graphics, versions of which can be found in pretty much every programming language.
In this lab, you will program a Java version of the robot to draw some simple images. This will give you a chance to continue practice writing Java code and calling methods on Java objects.
This lab will be completed in pairs. You should review the pair programming guidelines before you get started.
- The basic idea: take turns "driving" and "navigating" as you complete the lab. I've included notes about a few good places to switch roles in the lab writeup.
Objectives
- To practice writing and running Java code.
- To practice calling methods on objects, and passing parameters to those methods.
- To begin working with numbers and variables.
- To practice writing methods
Necessary Files
You will need to download and extract the Turtles BlueJ project from the
Turtles.zip file.
This project includes Turtle
class that has already been written for you, so you have a Turtle to work with. You will need to create (simple) classes as well.
- Remember to extract the BlueJ project from the zip file, and then delete the zip file!
The Turtle
-
The turtle class is relatively simple to use in a program--it works a lot like the Shapes from the previous lab. You will first need to instantiate a
Turtle
object using the following code:
If you do this (or instantiate the object by right-clicking in BlueJ), you should be able to see the Turtle on a canvas:Turtle turtle = new Turtle();
-
You can tell the turtle to move forward with the
forward()
method, and you can tell it to turn with theright()
andleft()
methods. If you don't want the turtle to draw a line when it moves, use thepenUp()
method to raise the pen (and remember to usepenDown()
to put it down again!) -
In code, you can call methods on the turtle by using dot notation---the name of the object, followed by a period (a dot), followed by the name of the method.
-
Remember that methods may (or may not!) have parameters: values that go inside the parentheses. Remember that parameters just include the value, not the data-type. So if you want to tell the turtle to move forward 20, you say
turtle.forward(20);
, not turtle.forward(int20); If you're not sure whether to include parameters or what to include, check the documentation below or the method comment that appears when you select a method in BlueJ:(1) tells you that the parameter is going to be a double (a number that can have a decimal point), (2) tells us what that number means (in this case, that it is the distance in pixels), and (3) is where we enter the parameter.
-
Remember that methods may (or may not!) have parameters: values that go inside the parentheses. Remember that parameters just include the value, not the data-type. So if you want to tell the turtle to move forward 20, you say
-
You can (and should!) experiment with the turtle by right-clicking on the Turtle class and creating a new instance in BlueJ, or by using the instantiation code in either a class or in the codepad.
- Helpful hint! After you create the turtle, make sure to click on the BlueJ window to give it focus again; this will make it easier to select methods from the object bench!
- Note that you can create multiple turtles and they will all show up on the same window; remember to give them different names!
Below is a list of some of the methods that the turtle supports:
/** * Moves the turtle forward the specified distance (in pixels) */ public void forward(int distance) /** * Turns the turtle to the left the specified number of degrees */ public void left(int angle) /** * Turns the turtle to the right the specified number of degrees */ public void right(int angle) /** * Puts the turtle's pen down. */ public void penDown() /** * Lifts the turtle's pen up. */ public void penUp() /** * Sets the color of the turtle's pen, using the name of the color. Can either be: * "red", "green", "blue", "cyan", "magenta", "yellow", "pink", "gray", "black", "white" */ public void setPenColorByName(String color) /** * Sets the turtle's speed. 0.5 is default, 1 is fastest, 0 is slowest. */ public void setSpeed(double speed)
Assignment Details
Drawing 1: A Bowtie
-
Let's start by drawing something following the instructions below (this is the same process you used in the last lab):
Create a Turtle object named myTurtle Set myTurtle's pen color to "blue" Turn myTurtle left by 90 degrees Move myTurtle forward by 300 Turn myTurtle right by 135 degrees Move myTurtle forward by 425 Turn myTurtle left by 135 Move myTurtle forward by 300 Turn myTurtle left by 135 Move myTurtle forward by 425
That should be straightforward, but incredibly tedious to do by hand. Can you image how long it would take to draw something more sophisticated than a bowtie? Or what happens if you screw up?! Luckily, we can program the computer to do all that work for us.
-
Go back to the BlueJ project window and create a new
Bowtie
object in the workbench. When you right-click to view it's methods, you'll notice that it doesn't have any! So let's add a method: double-click on the Bowtie class to view the source code. As you can see, besides the class declaration and a few comments, not much has been provided for you (thanks Joel). -
First, fill in your names next to the
@author
tag (e.g.,@author Joel & David)
, and add in a quick comment describing the class. -
Now the fun part: you need to create a new method for the object. We'll call it
draw()
:/** * REPLACE THIS: Fill in a brief bescription of what method does */ public void draw() { //add statements to draw the bowtie here }
- The first few lines are a block comment that describes the method. Recall that comments are completely ignored by the Java compiler, so they only serve the purpose of informing people reading the code. Go ahead and fill in a brief sentence about what this method will perform when it is called.
-
The next line is the all-important method signature. It says that the method is:
-
public
and so is visible to users. -
void
and so will not return any values -
draw()
is the method's name, followed by a list of parameters. In this case, no parameters are required.
-
- Everyelse is known as the method body. There is where the algorithm goes--the step-by-step instructions how to solve the problem!
- This is a good time to switch drivers!
-
The body of the
void draw()
method should run the algorithm you just executed. This means you'll need to translate from the natural language description to the Java language description.-
To instantiate (create) a new object of class
ClassName
calledobjectName
, you can write:ClassName objectName = new ClassName();
-
To call a method
methodName()
on an object, you can write:objectName.method(param1, param2, ...);
where
param1, param2, ...
are the parameters separated by commas. Note that the parameter list may be empty.
-
To instantiate (create) a new object of class
-
To get a feel for the translation process, we've gotten things started for you by providing code to create the Turtle and set its color. Your job is to complete the remaining translation and finish filling in the
draw()
method.Algorithm in Natural Language Algorithm in Java (unfinished) Create a Turtle object named myTurtle Set myTurtle's pen color to "blue" Turn myTurtle left by 90 degrees Move myTurtle forward by 300 Turn myTurtle right by 135 degrees Move myTurtle forward by 425 Turn myTurtle left by 135 Move myTurtle forward by 300 Turn myTurtle left by 135 Move myTurtle forward by 425
Turtle myTurtle = new Turtle();
myTurtle.setPenColorByName("blue");
Helpful hint! Try writing only a few method calls at a time, and then compile and test your method. Get used to this "write a little; test it; write a little more" workflow---it will make your life much easier in the future!
-
After you've implemented the method and successfully compiled your program, instantiate a new
Bowtie
object and call itsdraw()
method. The drawing should automatically appear, and you've just completed your first method in Java!
Drawing 2: Your Initials
-
For the second drawing, you should program the turtle to draw out the first initials of you and your partner. So if Joel and David were working together, we'd program the turtle to draw
J + D
- This is a good time to switch drivers!
- You should write this program in a new class to represent your drawing. Click on the New Class button and name your class
InitialDrawing
. -
You'll need to delete most of the sample code that BlueJ provides--everything except the class declaration. You'll then need to add in a
public void draw
method declaration. Your class should thus look like: -
Inside the body of the
draw()
method, you'll need to instantiate a newTurtle
object; see the above section for details. You can then use BlueJ to create a new red InitialDrawing object box, and then right-click on that to call yourdraw()
method. - You should program your turtle using the same tricks and techniques you worked on for drawing the bowtie. Be sure to write a bit of your code, then compile and run to test your program!
- You are welcome to get creative with this--using different colors, decorations, etc. But make sure you have time to complete the whole lab!
Drawing 3: The House
-
Your final task will be to write a program that instructs a turtle to draw a simple picture of a house:
- This is a good time to switch drivers!
- You should write this program in a new class to represent your house drawing. Click on the New Class button and name your class
HouseDrawing
. -
The pitch (or angle) of the roof should be 45 degrees, with the tallest point being lined up to the center of the house. Turning the turtle in the right directions should not be a problem, but calculating the distance that needs to be drawn can present some challenges. If you go too far or stop too short (see below), the pitch will be skewed to one side:
The trick to solving this problem is to observe that the side of the roof is the hypoteneuse, or the longest side (C), of a right triangle, where the other two sides (A) and (B) have equal length:
Luckily, we know the Pythagorean Theorem. Can you use it to solve for the hypotenuse? You will need to get the square root of a number, and Java provides this useful method:
whereMath.sqrt(n)
n
is a number. You can use this to calculate the length of the roof, and then plug that number into theforward()
method as follows:double roofLength = Math.sqrt(???); //this is a double because it may not be a whole number turtle.forward((int)roofLength);
(int)
in front of the roofLength to turn it back into a whole number that theforward()
method expects).
Submission
-
Make sure both of your names are on the classes you've created (
HouseDrawing
andInitialDrawing
). If your names aren't on your assignment, we can't give you credit! -
Right-click on the project folder you downloaded, then:
- If using Linux, select Compress...
- If using Windows, select Send to and then Zip file
- If using Mac, select Compress ... items
This will let you take the selected folder (or files) and generate a new compressed
.zip
file. -
Navigate to the course on Moodle
(Lecture Section A),
(Lecture Section B).
Upload your
.zip
file to theLab B Submission
page. Remember to click "Save Changes"! You may submit as often as you'd like before the deadline; we will grade the most recent copy.- Only one partner needs to submit the assignment!
- While you're on Moodle, remember to fill out the Lab B Partner Evaluation. Both partners need to submit evaluations.
Extensions
An OPTIONAL note about color:
-
Color is actually a complex topic in computer science; colors can be represented in lots of different ways. In the previous assignment, we used Strings (like "red") to talk about colors. That option is available here, using the
setPenColorByName
method. -
However, in Java, we more often use a previously-written class called
Color
. So the color red is represented by a particularColor
object---in Java almost everything is an object! So we can call thesetPenColor()
method and pass it a Color object instead of a String! -
In order to use this class, you need to tell Java that you want it (otherwise Java is lazy and won't include it). To do this, include the line:
This tells Java to include the class, which has the full name ofimport java.awt.Color
java.awt.Color
. We put this line at the very top of the file for the class where we want to use Color objects. -
Java has already created a lot of
Color
objects for you to use. These objects are referenced with the formColor.RED
orColor.BLUE
---note that the name of the color is in all capital letters (these are called constants; we will talk about them later). All of the colors we have used so far are available in this way---a full list can be found here. -
Finally, if you want to use this method to specify a color using the BlueJ interface (such as when you right-click on the method to call it), you'll need to use the full name of the Color object. For example:
java.awt.Color.RED
(note that this is one of the few instances we will use full names for classes). - Or you can just use the
setPenColorByName()
method, which lets you pass in the color name as a String.
Grading
This assignment will be graded out of 10 points.
- [2pt] Your Bowtie class has a
draw()
method that draws a Bowtie - [1pt] You have created a new
InitialDrawing
class with a draw() method - [2pt] Your InitialDrawing class can draw your initials wth a plus sign
- [1pt] You have created a new
HouseDrawing
class with a draw() method - [1pt] Your HouseDrawing class draws a picture of a house
- [1pt] Your house drawing has a roof sloped at 45 degrees
- [1pt] All classes have class comments (and author names!) filled in
- [1pt] Submitted a lab partner evaluation