CS 261 Lab F - Fractal Art
Due Wed Mar 05 at 9:00am
Overview
A fractal image is an image in which every small subsection is the same as the larger image it is a part of--if you keep zooming in on the image, you just keep seeing the same thing. These images are defined recursively--each piece of the image contains a copy of itself, and so on.
For example, consider the simple sideways H (It's an H, not an I!) shape labeled Step 1. If you draw similar, but smaller, H shapes at the end of each leg, you get the second shape (Step 2). Continuing that process until the line segments are too small to draw gives you the final shape (Step N).
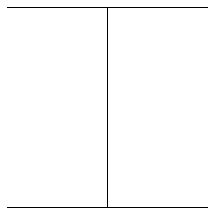
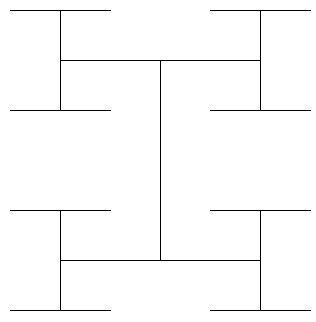
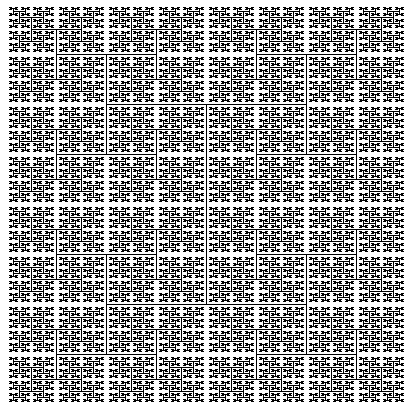
Although this is just a simple example (less than a dozen lines of code), more complicated images can be just as easy. Indeed, simple recursive rules like this can produce all kinds of amazing artwork.
In this lab, you will write programs to draw a pair of simple fractal images. You will draw one of either the above H-Tree or the Sierpinski Triangle, and one of either a fractal tree or the Koch Snowflake.
This lab will be completed in pairs. Review the pair programming guidelines! NOTE: although this lab may lend itself to simply splitting the work in half, you should follow pair programming practices and together write the code for both fractals!
Objectives
- To practice with recursion
- To draw pretty pictures
- To demonstrate you can use abstract classes
Necessary Files
You will need to download the copy of the
FractalLab.zip file.
This file contains two classes you will need to import into Eclipse. DrawableFrame
is a JFrame that you can draw on, such as we have used before. FractalDrawing
is an abstract class that you should use as the basis for your own drawings. This class provides functionality to initialize the frame, and provides you with a Graphics object that you can draw on. Do not modify either of these classes, simply use their methods! If you find that you're modifying them to make your code work, then you're doing it wrong!
The FractalDrawing
class also has a couple of helpful methods you will want to use. Calling show()
will make your drawing so far appear in the frame. Calling wait()
will make your program "pause" for a moment. You can use this to slow down the drawing so that you can see what's happening. On my computer, a pause time of 0 milliseconds is instantaneous, and pause time of 5 milliseconds look good on my machine, a pause time of 100 looked good to Dan. Your mileage may vary.
Details
- You will need to make two separate classes, one to draw each of your fractals. These classes should extend
FractalDrawing
. You will also need a Tester to start each of your fractals. -
Each of your classes will need to override the
drawFractal()
method. In this method, you should call helper methods to draw your fractal.- Consider what is happening at each step, and name your helper methods after that.
- Think about what values will be different at each step in the drawing: these will be your parameters.
-
Recursion can be tricky. It's a pretty abstract concept and it can be frustrating. But it is really cool when it works. Remember: A recursive method calls itself with a smaller problem and returns when the problem is small enough to be "easily solved".
- None of the fractal methods require more than a dozen or so lines of code (depending on how fancy you want to get with color, etc.). If you fine your method is getting much longer than that, take a moment to convince yourself you are on the right track!
- Hint: Write the "base case" draw function first, test that, then add in the recursion (so that at least you know your drawing is correct!) Hint 2: It is an excellent debugging strategy to print out the arguments given at the start of each call to the recurive method (e.g., make the first line of the method a printout that says "I am X method called with args A B C"). This can help you follow what is going on.
- Remember: you can use the
wait()
method to slow down your drawing and see what is happening. - When you are finished, make sure your code is well-documented: you do not need to include full Javadoc comments (though including a comment labeling your parameters will likely make your life easier) but you should have plenty of in-line comments to explain how your recursive methods work!
- Double-check that your fractals show up correctly and look good, and that your names are on the top of your classes, before submitting your new classes to the submission folder.
- Fill out the lab partner evaluation survey after you turn in your work!
Submitting
Submit your two fractal classes to the LabF submission folder on hedwig. Make sure you upload your work to the correct folder! The lab is due at the start of class on the day after the lab.
Remember to fill out your lab partner evaluation!
The Fractals
The H-Tree
More information about the H-Tree can be found here.
The Sierpinski Triangle
More information about the Sierpinski Triangle can be found here.
The Fractal Tree
More information about the fractal tree can be found here.
The Koch Snowflake
More information about the Koch Snowflake can be found here.
Extensions
You are welcome to implement more than one of these fractals if you have time. There are lots of other amazing fractals out there, including the infamous Mandelbrot Set
Grading
This assignment will be graded based on approximately the following criteria:
- You have implemented your fractal classes by extending the abstract FractalDrawer [10%]
- You have implemented either the H-Tree or the Sierpinski Triangle [30%]
- You have implemented either the Fractal Tree or the Koch Snowflake [40%]
- Your code is well documented and follows the edicts of good style [15%]
- You completed your lab partner evaluation [5%]