CS 315 Homework 1 - Android and Generative Art
Due Fri Sep 13 at 11:59pm
Overview
This assignment will give you a chance to get started with Android and graphical drawing, as well as to think about the design and implementation of graphical user interfaces.
You will be making a simple application that displays some form of Generative Art---a (generally 2D) drawing that is algorithmically defined by some set of inputs. For example, the fractals you may have drawn in 261 could be considered generative art: you define some set of parameters and these are used to generate a picture. In addition to drawing a picture, your application should provide a graphical user interface that allows the user to control the parameters of the generated art. For example, if my program displayed a fractal tree the user could select different colors for the branches and leaves, specify the depth level, and adjust the branching angles.

The drawing can be as simple or complex as you like (as long as it can be manipulated). Be inventive and creative with this! Come up with something neat and implement it in code. This is a chance for you to play around with drawing and the Android platform, to get used to the development system we will be using.
Some ideas for generative art genres:
- Spirographs or similar functions (note the "See also" topics at the bottom of the link)
-
Classical fractals, such as the
Koch Snowflake
or even the
Mandelbrot Set
- Note that recursive calls may have a noticable speed impact on your drawing when working in the resource-limited Android context. If possible, write fractals iteratively!
- Similarly, a simple Space-filling curve
- A simple L-system
- Metaballs or other blobby substance (this is one of my favorite forms).
- Random artwork
- If you are feeling bold, you might try something with animation or particle effects. See for example here here or here. Really HTML5 demos in generally may be a good source of inspiration.
- You might also look at things such as Screensavers (e.g., here for more ideas).
This assignment should be completed individually.
Objectives
- Install and become familiar with the Android development environment
- Become familiar with Android program structure, including Activities, Views, layouts, and resource organization.
- Specify an interactive graphical user interface using an XML layout specification
- Review a Java-based drawing API and create pretty pictures!
- Consider usability in the development of a graphical user interface
Necessary Files
You will need to install the Android SDK in order to create your application.
You will also want to download and extract a copy of the
starter code.
This basic activity includes all the setup you should need to begin drawing. Once you have the SDK installed, you can load this starter code by selecting File > New > Project > Android > Android Project from Existing Code
. Note that the zip file also contains a README.txt
file that you will need to fill out.
Details
This assignment is fairly open-ended; you are welcome and encouraged to be as creative as you'd like! However, there are a number of steps you should follow to get started.
The Development Environment
The first---and possibly most frustrating step---will be getting the Android development environment setup.
-
The first thing you should do is
install the Android SDK.
This should be installed on the machine in Th409, but installing on your own machine is also a good idea. Do this immediately! And let me know if you have any problems.
- Be sure to also install the API for Android 4.2.2 (API 17). This will be our target build level for the class (while it is possible to build for Android 4.3, we're going to work with Android 4.2.2 for this course. Android is backwards compatible, and you shouldn't notice the difference).
-
You'll also want to set up the Android emulator by creating an
Android Virtual Device.
The emulator will act as our runtime environment for the rest of the class. I will be testing this program on a virtual Nexus 4 (a high-end phone, not a tablet), so you should make a new AVD to reflect this. In Eclipse, select Window > Android Virtual Device Manager, then select select New. You should make a virtual device with the following configuration:
- Be sure and select the API level 17 (on my machine it wants to default to API 16).
- Also be sure and select Use Host GPU; we will need this for doing OpenGL ES work later in the semester.
- SUPER HELPFUL ADVICE: Once you've started the emulator, do NOT close it between application launches! You can hit the "Home" button (in the middle) to exit your app, and by selecting "Run" in Eclipse an updated version of your app will install and launch automatically. Starting the emulator can take a while, so the fewer times you have to do that, the better!
- Once you have your development environment all set up, I recommend you work (or at least skim) through the Getting Started tutorial to make sure your installation is working and to start getting the basics of the Android platform.
The Starter Code
Once you've got your development environment working, go ahead and import the starter code (you do not need to build an app from scratch). Open open the MainActivity.java
file from the src
folder and you should be able to see something like this:
Although there are a lot of pre-generated files in Android, there are only a few you should need to deal with:
-
src
folder This is where your Java code goes.- You'll note that the project has a package name "cs315.jross.hwk1". The first thing you should do is rename the package so that it at least has your name in it (I'm forgoing the normal edu.pugetsound.name format for the sake of typing). The easist way to rename this is to right-click on the project folder, select Android Tools [near the bottom] > Rename Application Package. If you don't do this, you might find yourself needing to change the name in other random files (like the manifest; see below).
-
There are three classes included in the starter code:
MainActivity
which is the "Activity" (think of it as the JFrame) for your application;DrawingView
which is a simple but less-optimized starter code for drawing, andDrawingSurfaceView
which is a more optimized (runs in its own thread) starter code for drawing. I've included comments explaining what each piece does; be sure and look at the Canvas and Drawables guide as well as the Custom Drawing examples for more details.
-
layout / activity_main.xml
This folder contains the XML-specified layouts for your application. See
UI Overview
for more details. This is basically where you specify what Views (similar to JComponents in Swing) go where. There are a lot of options you can include here, but go slowly--it's easy for things to break! The current layout includes the custom drawing View, as well as a button (as an example).
- Note that you can change between the simpler DrawingView and the faster DrawingSurfaceView by changing the entry in this xml file so that layout includes a different element!
-
values / strings.xml
Android has a pretty and complex system for organizing resources (data and assets you may want to use). See
Resources Overview
and
Accessing Resources
for more details. The
strings.xml
resource is one place to put constant display strings in your program. This is intended to make it easy to modify an apps appearance or support internationalization. For our purposes, it means that if you need to modify a String (like the text on a button), the value of that String might be initially defined in this file. Note that you can also modify things like button text programmatically. -
AndroidManifest.xml
This is the manifest file for your application. It includes all the basic information about what your program does, how it works, what functions it requires, etc. For this assignment you should not need to modify this file, but it's a good thing to look over. Note that it includes things like
"@string/app_name"
, which is a value defined in thatstrings.xml
and gives our application a name. - README.txt This is not an Android file, but a CS315 file that you will need to fill out!
Drawing a Picture
Given the starting code, drawing a picture should be very similar to drawing a graphical system in Java using Swing (JFrames) and Graphics2D. You can either set individual pixels in a Bitmap (basically a BufferedImage) or use built-in methods from the Canvas (basically a Graphics2D object). Be sure and look at the documentation for how to use these classes and what methods are available!
- Note that some classes that are normally found in Java SE are different in Android. For example, the Color class is much more simplistic and interger based.
- Paint is somewhat similar to the Java SE Paint, but stores more information. Note that Color is not a Paint.
- If there are any other confusing differences, let me know so I can update this page!
Adding Interaction
You will also need to add interactive input controls to your application so that the user can modify the generated art. Be sure and read the Input Controls guide for details.
You will need to specify this layout in the layout xml file. This is the proper way to do things in Android, rather than forcing the layout in code!
- The assumption is that you'll use basic interactive elements, such as Buttons Toggles or Selection Boxes to give users a small amount of control. (Note that Android does have Sliders which can make for nice interactions). These should be fairly simple to implement; use the existing Button as a template!
- You are welcome to add in Touch Gestures but they are not required for this assignment (we'll be dealing with touch gestures for the next homework!)
- Your artwork should have a minimum of 3 (three) interaction points and control widgets, and the more the better!
Be sure and consider the principles and guidelines we've covered in class and in the readings when designing your application and interactions.
- You should strongly consider sketching out what your app is going to look like before you implement it! Perhaps make a wireframe or powerpoint mock-up of your application. A tool like mockingbird can be helpful for this!
- Remember, a significant part of this assignment is designing an effective user interface. Give this some forethought before you jump in and start coding it!
Some Advice
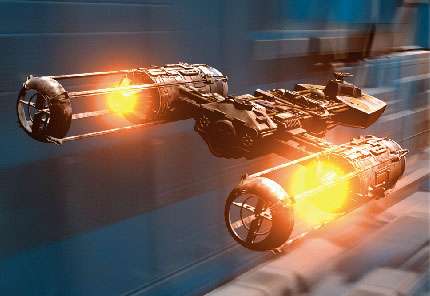
This first assignment is sizable and open---that means it is easy to get lost or bogged down. In the words of Gold Five: Stay on target! Remember the goals of the assignment: get Android up and running, draw a picture, and give it a nice interface. Your program does not need to be incredibly complex, it should just look good! Once you've got the basics down, then you can try adding extra complexity or functionality.
This is also a fairly self-directed assignment; the documentation is generally readable (though often provides too much information), but you are expected to read that documentation and learn from it. That means you need to take ownership for your own learning. If you don't know how to do something, try looking it up! And if you can't figure it out, come ask for help.
Also, be sure and get started early! Programming always takes longer than you think, and needing to learn the new set of tools and classes is going to slow you down. Don't leave this until the last minute.
Extensions
Once you've got the basics put together, go all out! Exceptional, creative submissions makes for a happy professor, and a happy professor may give you extra credit.
Submitting
BEFORE YOU SUBMIT: make sure your code is fully documented and functional! If your code doesn't run, I can't give you credit! And yes, you should include comments explaining what your code and methods do! Exclamation point!
Upload your entire project (including all the src, resources, layouts, libraries, etc) to the Hwk1 submission folder on hedwig (see the instructions if you need help). Also include a filled-in copy of the README.txt file in your project directory!
The homework is due at midnight on Fri Sep 13. Don't be friggatriskaidecaphobic and be late!
Grading
This assignment will be graded based on approximately the following criteria:
- Includes a working Android application with user interaction [10%]
- Displays generative artwork [30%]
- Includes at least 3 working points of interaction for the user to control the art [30%]
- Application demonstrates good GUI design principles [20%]
- Code is documented and understandable [5%]
- README is completed [5%]